By: Hristo Hristov | Updated: 2022-04-14 | Comments | Related: > Python
Problem
So far in our Python tutorial series we have covered many of the basics of working with the Python programming language, e.g., data types, using functions and control flow management with conditional logic and loops. As a scripting language, Python also offers many built-in functions that are helpful to the programmer. While it is difficult to enumerate all of them, there are some which are considered important and popular.
Solution
This tip examines 15 important functions in Python. We are not saying the "most" important – that is rather subjective. On the other hand, all these functions are built-in and do not require importing any additional packages – you can use them right away in whichever Python distribution you have running. Trying out these functions is also a good way to get familiar with Python’s workings. Let us kick it off in alphabetic order with examples outlining the function name, function definition, function parameters, default values, sample code and return statements.
1. abs
abs(x)
Returns the absolute value of a given number. The parameter can be an integer, floating point number or a complex number within the parentheses. Here is the syntax:
num = -3 abs(num)
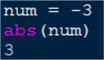
2. all
all(iterable)
With an input parameter of an iterable, this function returns True
if all elements of the input are true, otherwise it returns False
.
Check out the following example:
my_list = [1,2,3] my_set = {0,1,2} my_tuple = (0,1) print(all(my_list), all(my_set), all(my_tuple))

The return value 0 is naturally treated as False
. Therefore, we get
False
as outcome from the latter two calls. Passing an empty collection
to all
will also return False
. On the other hand, the
variable my_list
technically does not contain any False
values, therefore all
returns True
for it.
3. any
any(iterable)
Like all
but returns True
if at least one variable
of the iterable input argument is True. Using the same variables declared in the
example for all
, this is the result:
print(any(my_list), any(my_set), any(my_tuple))

4. dir
dir([object])
This helpful function can work in two ways: without and with arguments. If you just call the function like this with no arguments:
dir()
you will get a list of the names in the current local scope. This local scope for me right now is the Python IDLE 3.10. shell. I get a bunch of the default attributes available and all variables I have declared in this session, e.g., "columns", "my_set", etc.:

If you are navigating a custom scope, dir
can help you find your
way round it. You can directly invoke those attributes:
print(__name__) print(__doc__)

The name of my module is __main__
and there is no docstring for
it. Additionally, you can call dir
with an argument. In that case,
it will return a list of the attributes of the argument object. For instance, this
is how we get all attributes and methods of the dictionary class:
dir(dict())

In this way, dir
is helpful to show you what attributes (and in
this case also methods) the object has. Some of them should look familiar, as we
presented them in the tip on
Python complex data types when the dictionary type was discussed.
5. enumerate
enumerate(iterable, start=0)
In a nutshell, enumerate allows you to generate a sequence containing an index for each of the elements of the passed argument. Therefore, the argument must always be an object supporting iteration, e.g., list, dictionary, or custom object. By default, the enumerate function call returns an enumerate object:
my_list = ['SQL', 'PowerBi', 'Azure'] enumerate(my_list) <enumerate object at 0x000001F4F2ACA6C0>

You can cast the enumerate object to something that you can make better use of, such as a list, dictionary, set or tuple:
list(enumerate(my_list)) dict(enumerate(my_list)) set(enumerate(my_list)) tuple(enumerate(my_list))
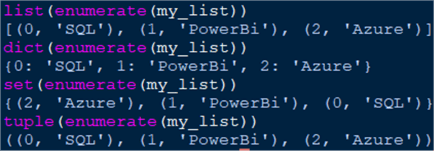
The start index defaults to zero but can be set to a different number using the
start
argument. In the future we will examine more closely how enumerate
can be helpful, for example generating multiple plots on one figure with matplotlib.
6. filter
filter(function,iterable)
Filter accepts a function as the first argument and an iterable as second. This
combination allows you to take only those elements from the input iterable for which
the function returns True
. For example, let us make a list of topics,
a function to match a certain topic and pass all of this to a filter
expression:
my_list = ['SQL', 'MySQL', 'PowerBi', 'Azure'] def match_topic(topic): result = True if 'SQL' in topic else False return result filtered_result = filter(match_topic, my_list)
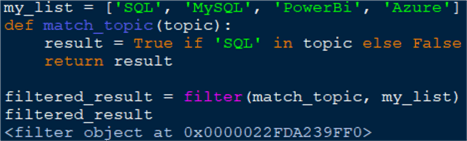
To get the results out of a filter expression it is enough to:
for x in filtered_result: print(x)
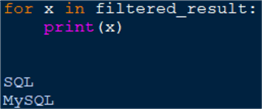
To tie things back to the tip on comprehensions, filter
is functionally
equivalent to the following list comprehension expression:
[item for item in my_list if match_topic(item)]
7. hash
hash(object)
Provide the hash value of an input object. Hash values are integers and are used to quickly compare values. An identical hash of two variables means the variables are equal. Hash accepts only hashable objects as input arguments, meaning only immutable types such as string:
my_str = 'ABC' hash(my_str)
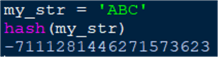
8. help
help([object])
Invokes the built-in Python help utility. Running help
in IDLE 3.10
looks like this:
help()
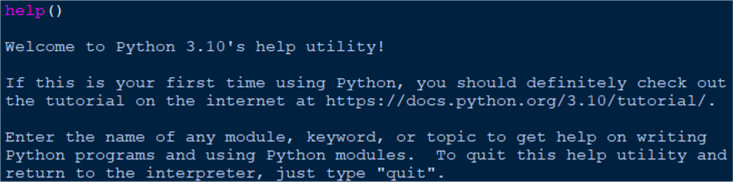
Additionally, I can pass an object name to the help function:
help(dict())
This action will display all built-in help documentation available in the module for that object (screenshot is abridged):
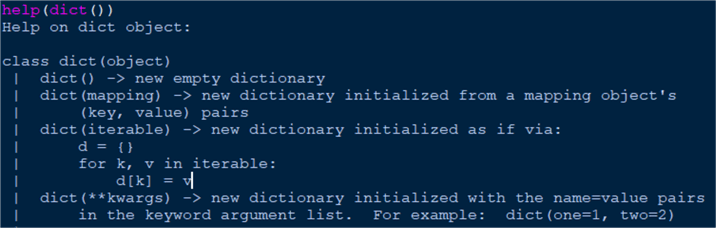
9. iter
iter(object[, sentinel])
This function brings us a step closer to differentiating between an iterable and an iterator object in Python. So let us quickly investigate that first (a special tip on that is also forthcoming).
An iterable is an object:
- whose elements you can access one by one with and index
- that implements the
__iter__
method which returns an iterator - that is allowed to be used in on the right-side of a for-loop expression:
for x in iterable
: - that implements the
__getitem__
method, used to access items from a list or dictionary entries.
An interator, on the other hand, is:
- A stateful object which keeps track of the last element returned during iteration
- Implements a
__next__
method that returns the next value in the iteration sequence, updates the state of the iterator and knows when it has reached the end of the iteration.
Let us try iter
out:
my_list = [1,2,3] my_iter_list = iter(my_list) next(my_list) next(my_iter_list) next(my_iter_list) next(my_iter_list)
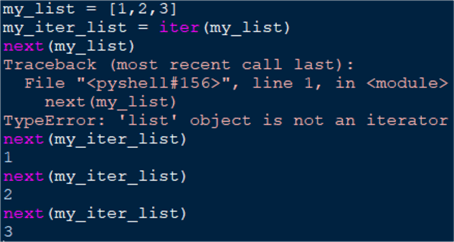
Notice that by default, list is an iterable but not an iterator. Therefore, using
next()
and passing the regular iterable to it will raise an error because
it does not implement the __next__
method. On the other hand, casting
the iterable to an iterator, we can take advantage of accessing its value on demand,
one by one.
iter
can also accept a sentinel value. This is a type of a stopper,
however if you supply it as a second argument according to the definition, the first
argument must a callable function (frequently called just callable). To illustrate
is best:
a = 1 def triple_number(): global a a = a * 3 return a my_iter = iter(triple_number, 81) for item in my_iter: print(item)
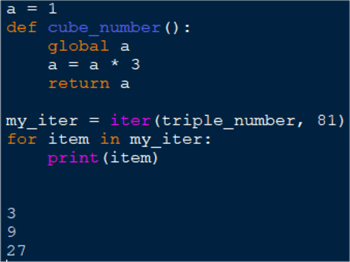
Starting with 1, we are tripling a number. We are instructing our new iterator
to terminate before the value of 81 (non-inclusive). Beware this can create a run-away
iterator if the sentinel value is wrong, e.g., a value of 80 will never be reached
if taking the cube of a number starting at 3. Such a mistake will cause iter
to continuously call the function and the program will never end unless manually
terminated.
10. len
len(s)
Returns the length of an object, provided that the object is a either a sequence (e.g., string, tuple, range) or a collection (e.g., dictionary, set).
my_str = 'SQL' my_dict = {1:'value1', 2:'value2', 3:'value3'} print(len(my_str), len(my_dict))

Accessing the elements of a collection index-wise generally calls for using
len
:
my_list = ['S', 'Q', 'L'] for index in range(len(my_list)): print(index, my_list[index])
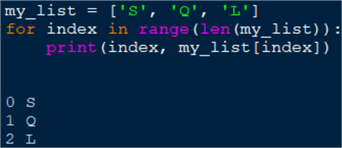
Notice how this use of len
emulates enumerate
but returning
the tuples one by one, instead of altogether.
11. map
map(function, iterable)
This is a powerful function which allows you to apply a function to every item
of an iterable. For instance, here is how to apply abs
to a list of
numbers:
my_numbers = [-2, 3, -6] my_map = map(abs, my_numbers) for item in my_map: print(item)
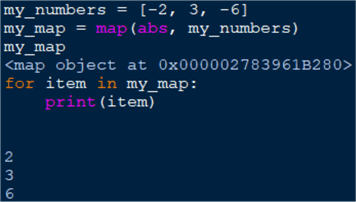
Map
returns a map object which is an iterator. Instead of
abs
, you can pass a function that you wrote.
12. pow
pow(base,exp[,mod])
Allows you to easily return base to the power of an exponent (exp
).
Optionally, you can pass a value for mod
to calculate the modulo division
of the result.
result1 = pow(3,3) result2 = pow(3,3, 5) print(result1, result2)
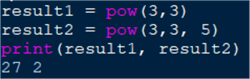
The first result is 27 (3 to the power of 3). The second one is 2, as 5 is contained 5 whole times in 27, giving a modulo division result of 2 (27 – 5 * 5 = 2).
13. print
print(*objects, sep=' ', end='\n', file=sys.stdout, flush=False)
This ubiquitous function allows you to display the result of your script. The
sep
argument allows you to pass a string as a separator for multiple
values. The end
argument enables you to pass a string to be used to
terminate the text sequence. By default, the file argument is sys.stdout which is
a file object serving as the default output for the interpreter. This output typically
is the current script window, however, you can set it to different places (e.g.
external file or OS shell). The flush argument allows you to close the buffer immediately
if needed.
topics = ['SQL', 'PowerBi', 'Azure'] print(*topics, sep='; ', end='\r\n')
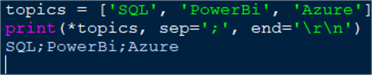
14. type
type(object)
This handy function allows you to return the type of an object. For example:
unknown_objects = [(),{},''] [print(type(x), end='\r\n') for x in unknown_objects]

However, according to the Python documentation it is recommended to use
isinstance(object, check)
for checking the type of an object:
unknown_objects = [(),{},''] [print(isinstance(x, list), end='\r\n') for x in unknown_objects]

In practice, isinstance
may be more helpful as it performs the check
for you, instead of just returning the target’s type like type
does.
15. zip
zip(*iterables, strict=False)
Accepts two or more iterables and iterates over their elements in parallel. The result is tuples of the items belonging to each iterable. Let's see how it works with this block of code:
levels = [100,200,400] topics = ['SQL', 'PowerBi', 'Azure'] published = [True, False, True] result = zip(levels, topics, published) [print(item) for item in result]
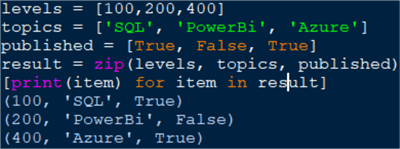
Here we have three lists of equal length. zip
pairs up every item
of every list with each other producing three tuples. Now let us examine what happens
if one of the iterables had more elements that the others. This is where the
strict
argument comes into play.
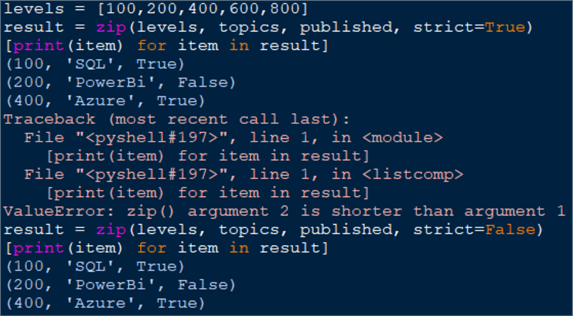
Re-declaring only the levels
variable is making it 5 elements long.
On one hand, with strict=True
, we do get an error saying the second
argument has fewer elements than the first. On the other hand, with strict=False
,
the error is suppressed, and we get a set of three tuples, which is the length of
the shortest list used as an argument. Using zip with the error suppression option
can be risky. Being difficult to spot, such a silent error can cause lots of headache
in further parts of the program.
Conclusion
In this tip we presented 15 valuable and handy functions helping you to become a better programmer. Be sure to check the Python code documentation for further guidance and comment on this article with questions.
Next Steps
Learn Python with me
About the author
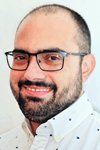
This author pledges the content of this article is based on professional experience and not AI generated.
View all my tips
Article Last Updated: 2022-04-14