By: Rajendra Gupta | Updated: 2024-05-02 | Comments | Related: > Python
Problem
How do you work with strings in Python programming? What built-in functions are available? What is the syntax? How can I get started as a beginner?
Solution
Working with strings is essential in programming code in any language. You might need to print specific outputs, give instructions and take user inputs. Let's explore how we work with strings in Python.
Suppose you have two variables that store the first_name and last_name, as seen below.
- first_name: Virat
- last_name: Kohli
Print Single Line Strings in Python
Let's start with an introductory message we want to print in Python. The message is "Virat Kohli is a famous cricketer." You can use a print() statement for the message.
print(first_name,last_name,'is a famous cricketer')
As shown below, by default, print statements add space between strings.
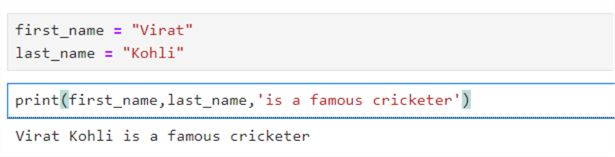
Now, let's change the message a bit. You need to print the message below.
- 'Virat Kohli' is a famous cricketer.
You can use the print statement below. In this method, we use a concatenate operator (+) and enclose the single quote within the double quotes to print the single quote.
print(" ' " + first_name + " "+ last_name + " ' " + "is a famous cricketer")

Alternatively, Python provides a print(f" ") statement for the formatted strings. This method is commonly known as F-Strings. Here, we use variable names inside the curly brackets {}
For our requirement, the F-string code is as follows. It uses double quotes to enclose the single quote string.
print(f" '{first_name} {last_name}' is a famous cricketer")

Let's take another example that prints the message below:
- "Virat Kohli" is a famous cricketer.
To print this message, we can use the single quotes to enclose the double quotes, as shown below.
print(f' "{first_name} {last_name}" is a famous cricketer')

What if the requirement is to print the string as "Virat Kohli" is a 'famous' cricketer?
We can use triple-double or triple-single quotes like the one below for this requirement.
print(f""" "{first_name} {last_name}" is a 'famous' cricketer""")
print(f''' "{first_name} {last_name}" is a 'famous' cricketer''')
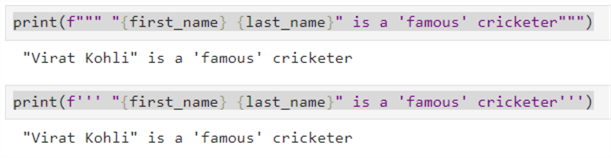
Print Multi-line Strings in Python
Sometimes, you might need to print a long sentence that requires several lines. To print these lines, you can use the following methods.
Triple Single Quotes
print('''Hi My Name is Rajendra You are learning Python ''')
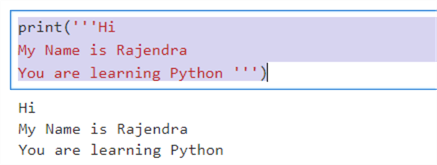
Triple-Double Quotes
print("""Hi My Name is Rajendra You are learning Python """)
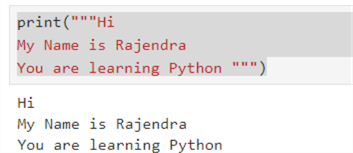
Parentheses and Single/Double Quotes
print("Hi \n" "My Name is Rajendra \n" "You are learning Python")
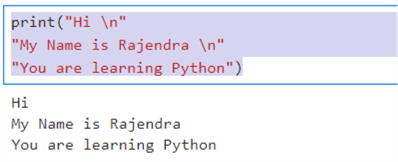
Dynamic Inputs (User Input) in Python
You can use the input() function to get a user input.
For example, once you run the code below, it asks you to enter the value for the name variable.
name = input()

But if you have multiple inputs from the user, it isn't easy to understand which parameter you are passing the value. Therefore, the input() function also provides functionality to print labels, as shown below.
name = input('Enter your name :')

Let's look at another example of user input of two numbers.
num1 = input('Enter the number 1: ') num2 = input('Enter the number 2: ') print(num1 + num2)
If we run this code with the num1 value as 5 and the num2 value as 2, it gives the following output. It did not perform addition. Instead, it concatenated the inputs. Why?
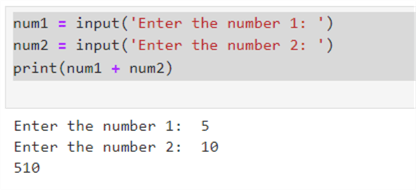
By default, the input function treats the user inputs as strings. We can verify it by checking the type of input values.
num1 = input('Enter the number 1: ') num2 = input('Enter the number 2: ') print(type(num1), type(num2))
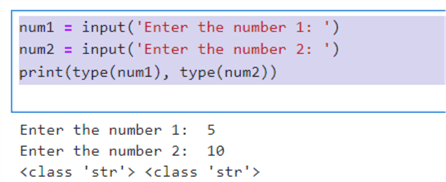
We need to convert the data type from a string to an int to perform the mathematical operation on the inputted data.
num1 = input('Enter the number 1: ') num2 = input('Enter the number 2: ') num1 = int(num1) num2 = int(num2) print(num1 + num2)
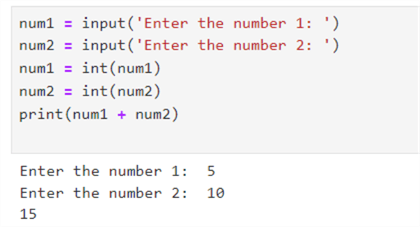
Similarly, if you give the input as a float, you must convert the values like the ones below before performing mathematical operations.
num1 = input('Enter the number 1: ') num2 = input('Enter the number 2: ') num1 = float(num1) num2 = float(num2) print(num1 + num2)
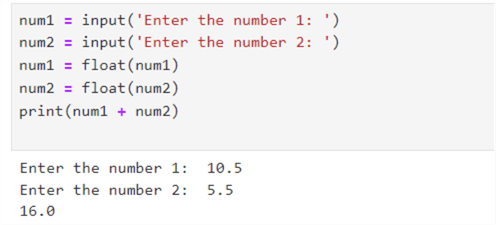
String Formatting Functions
Upper Function
Python upper() function converts all string characters into upper case.
name = "rajendra gupta" print(name.upper())
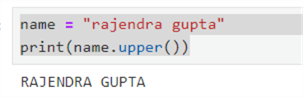
Lower Function
Python lower() function converts all string characters into lowercase.
name = "RAJENDRA GUPTA" print(name.lower())
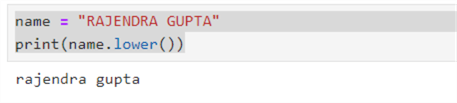
Capitalize Function
This function converts the first character of a string into upper case.
name = "RAJENDRA GUPTA" print(name.capitalize())
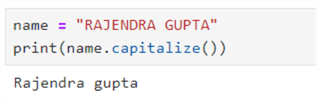
Title Function
This function converts the first letter of all words of a string into uppercase.
name = "you are LEARNING PYTHON on mssqltips" print(name.title())

Strip Function
The Python strip function removes any leading and trailing characters in the given string. By default, it removes the leading and trailing whitespace.
name = " you are LEARNING PYTHON on mssqltips" name.strip()

You can specify a character to remove from the string using the strip function.
Suppose we want to remove the characters ab from the given string:
- It removes the characters from the left side until it gets a mismatch. The example below removes characters ab until the first mismatch c occurs.
- It removes characters from the right side until it gets a mismatch. In the example below, it removes character ab until the first mismatch h occurs.
Note: It does not remove any character after a mismatch. Therefore, you get the output string with the character ab.
str = "abab cdef ab gh ab" str.strip('ab')
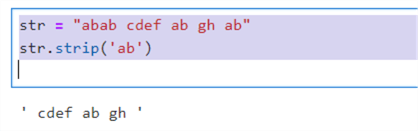
If you want to remove characters from the left side only, use the lstrip function.
str = "abab cdef ab gh ab" str.lstrip('ab')
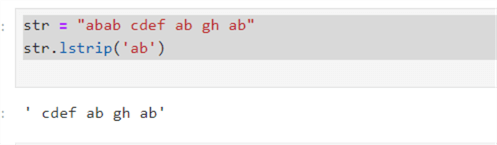
Similarly, use the rstrip function to remove characters from the right side.
str = "abab cdef ab gh ab" str.rstrip('ab')
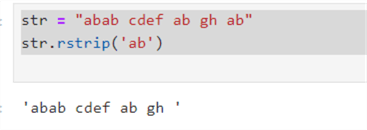
Split Function
It splits the Python strings based on the specified delimiter. By default, Python considers a space as a delimiter.
string = "Rajendra Gupta" name = string.split() name
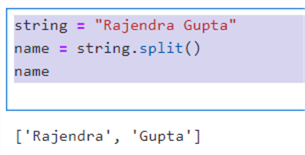
You can specify the delimiter, such as a comma, in the split function.
string = "John,Ross,Michael" names = string.split(",") names
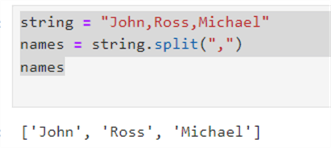
Replace Function
The replace() function replaces occurrences of a substring from a string with another substring.
string = "John Wichael" new_string = string.replace("Wichael", "Michael") print(new_string)
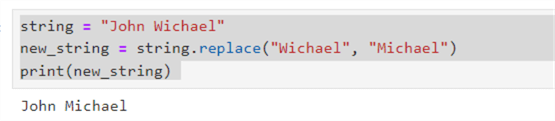
Next Steps
- Stay tuned for more Python tutorials in the upcoming tips.
- Explore existing SQL Server Python tips on MSSQLTips.
About the author
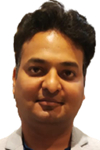
This author pledges the content of this article is based on professional experience and not AI generated.
View all my tips
Article Last Updated: 2024-05-02